- Visual Studio Code Hello World Vb.net
- Visual Studio Code Hello World Python
- React Hello World Visual Studio Code
Our first app is a 'Hello, World!' App that demonstrates some basic features of interactivity, layout, and styles. Begin by creating a new project in Microsoft Visual Studio. Create a Blank App (C/WinRT) project, and name it HelloWorldCppWinRT. Don't forget to watch part 2!!-how to start a project in MVS.how to output text.how to comment.
-->Client-side web parts are client-side components that run in the context of a SharePoint page. Client-side web parts can be deployed to SharePoint environments that support the SharePoint Framework. You can also use modern JavaScript web frameworks, tools, and libraries to build them.
Client-side web parts support:
- Building with HTML and JavaScript.
- Both SharePoint Online and on-premises environments.
Note
Before following the steps in this article, be sure to Set up your development environment.
You can also follow these steps by watching this video on the SharePoint PnP YouTube Channel:
Create a new web part project
Create a new project directory in your favorite location.
Go to the project directory.
Create a new HelloWorld web part by running the Yeoman SharePoint Generator.
When prompted:
- What is your solution name?: helloworld-webpart
- Which baseline packages do you want to target for your component(s)?: SharePoint Online only (latest)
- Where do you want to place the files?: Use the current folder
- Do you want to allow the tenant admin the choice of being able to deploy the solution to all sites immediately without running any feature deployment or adding apps in sites?: N
- Will the components in the solution require permissions to access web APIs that are unique and not shared with other components in the tenant?: N
- Which type of client-side component to create?: WebPart
The next set of prompts ask for specific information about your web part:
- What is your Web part name?: HelloWorld
- What is your Web part description?: HelloWorld description
- Which framework would you like to use?: No JavaScript framework
At this point, Yeoman creates the project scaffolding (folders & files) and installs the required dependencies by running npm install
. This usually takes 1-3 minutes depending on your internet connection.
When the project scaffolding and dependency install process is complete, Yeoman will display a message similar to the following indicating it was successful:
Important
NPM may display warnings and error messages during the installation of dependencies while it runs the npm install
command. You can safely ignore these log warnings & error messages.
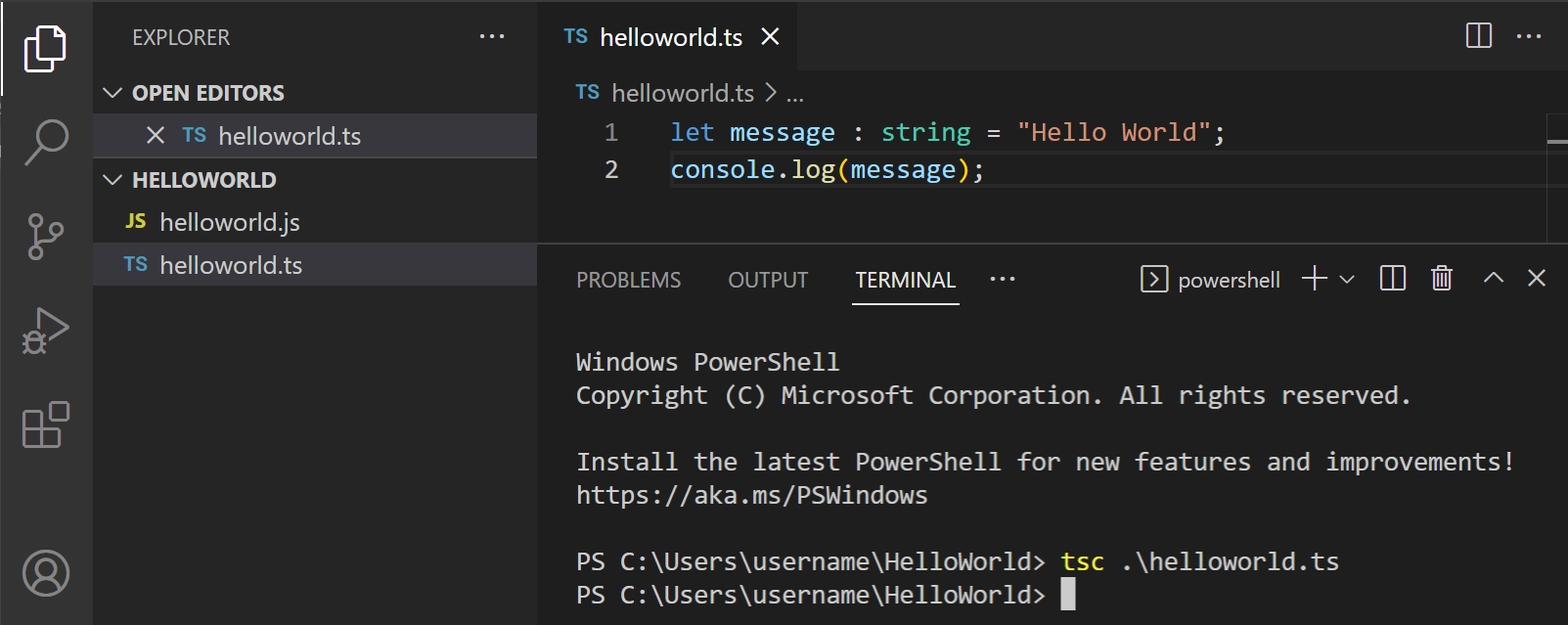
NPM may display a message about running npm audit
at the end of the process. Don't run this command as it will upgrade packages and nested dependencies that may not have been tested by the SharePoint Framework.
For information about troubleshooting any errors, see Known issues.
Use your favorite code editor

Because the SharePoint client-side solution is HTML/TypeScript based, you can use any code editor that supports client-side development to build your web part, such as:
The SharePoint Framework documentation uses Visual Studio Code in the steps and examples. Visual Studio Code (VS Code) is a lightweight but powerful source code editor from Microsoft that runs on your desktop. VS Code available for Windows, macOS, and Linux. It comes with built-in support for JavaScript, TypeScript, Node.js, and has a rich ecosystem of extensions for other languages (such as C++, C#, Python, PHP) and runtimes.
Preview the web part
You can preview and test your client-side web part locally on your workstation. The client-side toolchain uses HTTPS endpoint by default. Part of the Set up your development environment process included trusting the development SSL certificate included in the toolchain on your local environment. This is required so your browser will trust the certificate.
Important
Trusting the developer certificate is required. This is a one-time process and is only required when you run your first SharePoint Framework project on a new workstation. You don't need to do this for every SharePoint Framework project.
If you didn't trust the dev cert, follow the steps outlined on this page: Set up your development environment: Trusting the self-signed developer certificate.
Now that we've installed the developer certificate, enter the following command in the console to build and preview your web part:
This command executes a series of gulp tasks to create and start a local webserver hosting the endpoints localhost:4321 and localhost:5432. It will then open your default browser and load the workbench preview web parts from your local dev environment.
Note
If you're seeing issues with the certificate in browser, please see details on installing a developer certificate: Set up your development environment: Trusting the self-signed developer certificate.
If you're still seeing issues, see: SharePoint Framework known issues and frequently asked questions
SharePoint client-side development tools use gulp as the task runner to handle build process tasks such as:
- Compiling TypeScript files to JavaScript.
- Compiling SASS files to CSS.
- Bundling and minifying JavaScript and CSS files.

VS Code provides built-in support for gulp and other task runners. Select CTRL+SHIFT+B on Windows or CMD+SHIFT+B on macOS to debug and preview your web part.
The SharePoint Workbench is a developer design surface that enables you to quickly preview and test web parts without deploying them in SharePoint. SharePoint Workbench includes the client-side page and the client-side canvas in which you can add, delete, and test your web parts in development.
Use SharePoint Workbench to preview and test your web part
To add the HelloWorld web part, select the add icon (this icon appears when you mouse hovers over a section as shown in the image above). This opens the toolbox where you can see a list of web parts available for you to add. The list includes the HelloWorld web part as well other web parts available locally in your development environment.
Select HelloWorld to add the web part to the page.
Congratulations! You've just added your first client-side web part to a client-side page.
Select the pencil icon on the far left of the web part to reveal the web part property pane.
The property pane is where you can define properties to customize your web part. The property pane is client-side driven and provides a consistent design across SharePoint.
Modify the text in the Description text box to Client-side web parts are awesome!
Notice how the text in the web part also changes as you type.
One of the capabilities of the property pane is to configure its update behavior, which can be set to reactive or non-reactive. By default, the update behavior is reactive and enables you to see the changes as you edit the properties. The changes are saved instantly when the behavior is reactive.
Web part project structure
To use Visual Studio Code to explore the web part project structure
In the console, stop the local web server by terminating the process. Selecting CTRL+C on Windows or macOS.
Enter the following command to open the web part project in VS Code (or use your favorite editor):
Note
If you get an error when executing this command, you might need to install the code command in PATH.
TypeScript is the primary language for building SharePoint client-side web parts. TypeScript is a typed superset of JavaScript that compiles to plain JavaScript. SharePoint client-side development tools are built using TypeScript classes, modules, and interfaces to help developers build robust client-side web parts.
The following are some key files in the project.
Web part class
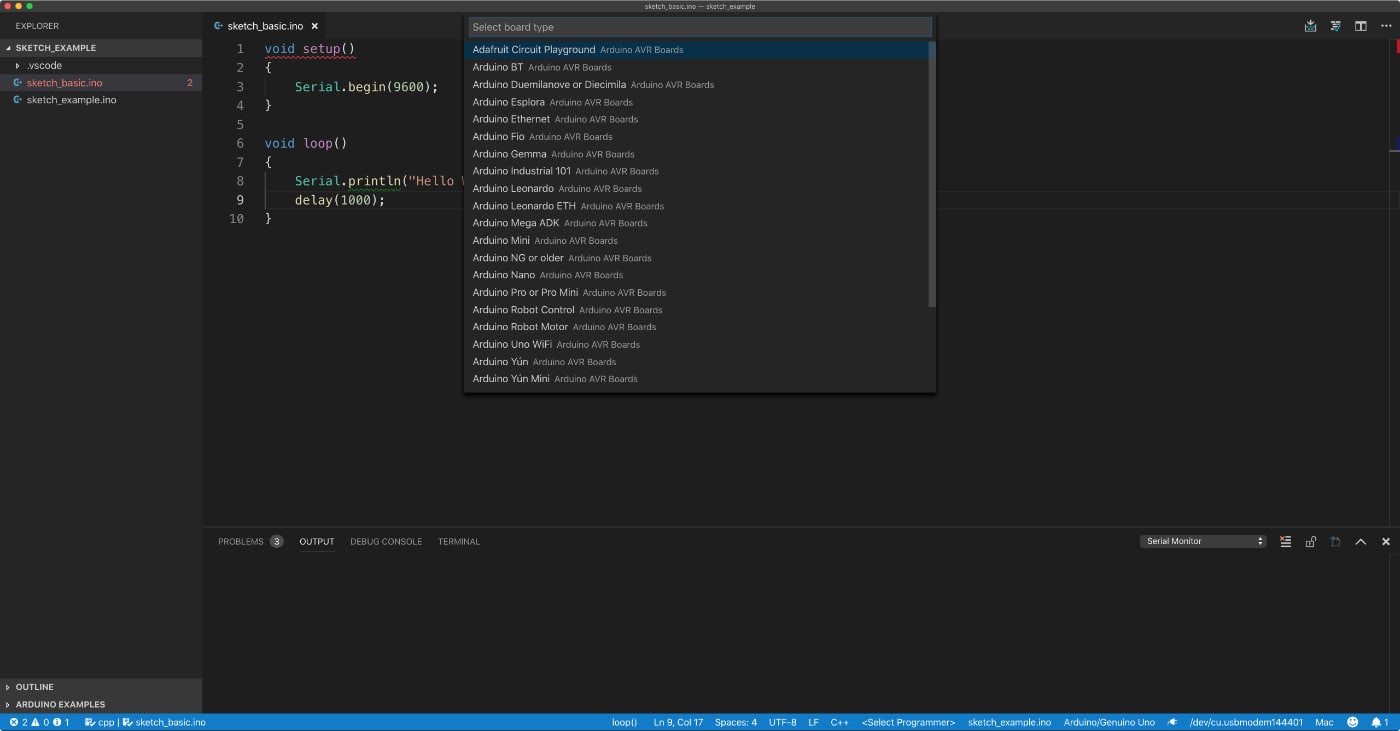
HelloWorldWebPart.ts in the srcwebpartshelloworld folder defines the main entry point for the web part. The web part class HelloWorldWebPart extends the BaseClientSideWebPart
. Any client-side web part should extend the BaseClientSideWebPart
class to be defined as a valid web part.
BaseClientSideWebPart
implements the minimal functionality that is required to build a web part. This class also provides many parameters to validate and access read-only properties such as displayMode
, web part properties, web part context, web part instanceId
, the web part domElement
, and much more.
Notice that the web part class is defined to accept a property type IHelloWorldWebPartProps
.
The property type is defined as an interface before the HelloWorldWebPart
class in the HelloWorldWebPart.ts file.
This property definition is used to define custom property types for your web part, which is described in the property pane section later.
Web part render method
The DOM element where the web part should be rendered is available in the render()
method. This method is used to render the web part inside that DOM element. In the HelloWorld web part, the DOM element is set to a DIV. The method parameters include the display mode (either Read or Edit) and the configured web part properties if any:
Visual Studio Code Hello World Vb.net
This model is flexible enough so that web parts can be built in any JavaScript framework and loaded into the DOM element.
Configure the Web part property pane
The property pane is defined in the HelloWorldWebPart
class. The getPropertyPaneConfiguration
property is where you need to define the property pane.
When the properties are defined, you can access them in your web part by using this.properties.<property-value>
, as shown in the render()
method:
Notice that we're executing an HTML escape on the property's value to ensure a valid string. To learn more about how to work with the property pane and property pane field types, see Make your SharePoint client-side web part configurable.
Let's now add a few more properties to the property pane: a checkbox, a drop-down list, and a toggle. We first start by importing the respective property pane fields from the framework.
Scroll to the top of the file and add the following to the import section from @microsoft/sp-property-pane:
The complete import section looks like the following:
Update the web part properties to include the new properties. This maps the fields to typed objects.
Replace the
IHelloWorldWebPartProps
interface with the following code.Save the file.
Replace the
getPropertyPaneConfiguration()
method with the following code, which adds the new property pane fields and maps them to their respective typed objects.After you add your properties to the web part properties, you can now access the properties in the same way you accessed the
description
property earlier:To set the default value for the properties, you need to update the web part manifest's
properties
property bag.Open HelloWorldWebPart.manifest.json and modify the
properties
to:
The web part property pane now has these default values for those properties.
Web part manifest
The HelloWorldWebPart.manifest.json file defines the web part metadata such as version, ID, display name, icon, and description. Every web part must contain this manifest.
Visual Studio Code Hello World Python
Now that we have introduced new properties, ensure that you're again hosting the web part from the local development environment by executing the following command. This also ensures that the previous changes were correctly applied.
Preview the web part in SharePoint's hosted workbench
React Hello World Visual Studio Code
SharePoint Workbench is also hosted in SharePoint to preview and test your local web parts in development. The key advantage is that now you're running in SharePoint context and you're able to interact with SharePoint data.
Go to the following URL: https://your-sharepoint-tenant.sharepoint.com/_layouts/workbench.aspx
Note
If you do not have the SPFx developer certificate installed, Workbench notifies you that it is configured not to load scripts from localhost. Stop the currently running process in the console window, and execute the gulp trust-dev-cert command in your project directory console to install the developer certificate before running the gulp serve command again. For more information, see Trusting the self-signed developer certificate
Notice that the SharePoint Workbench now has the Office 365 Suite navigation bar.
Select the add icon in the canvas to reveal the toolbox. The toolbox now shows the web parts available on the site where the SharePoint Workbench is hosted along with your HelloWorldWebPart.
Add HelloWorld from the toolbox. Now you're running your web part in a page hosted in SharePoint!

Note
The color of the web part depends on the colors of the site. By default, web parts inherit the core colors from the site by dynamically referencing Office UI Fabric Core styles used in the site where the web part is hosted.
Because you're still developing and testing your web part, there is no need to package and deploy your web part to SharePoint.
Next steps
Congratulations on getting your first Hello World web part running!
Now that your web part is running, you can continue building out your Hello World web part in the next topic, Connect your web part to SharePoint. You will use the same Hello World web part project and add the ability to interact with SharePoint List REST APIs. Notice that the gulp serve command is still running in your console window (or in Visual Studio Code if you're using that as editor). You can continue to let it run while you go to the next article.
